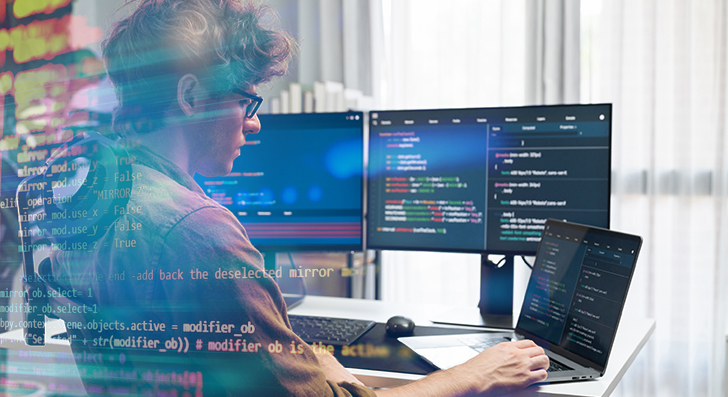
Scalability means your application can deal with growth—extra people, a lot more data, and more traffic—without the need of breaking. Being a developer, developing with scalability in your mind saves time and stress later on. Listed here’s a clear and simple guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on afterwards—it should be aspect of one's system from the beginning. Quite a few applications fall short when they improve quick mainly because the original layout can’t handle the extra load. To be a developer, you should Imagine early about how your technique will behave stressed.
Begin by coming up with your architecture to become versatile. Stay clear of monolithic codebases exactly where anything is tightly related. As an alternative, use modular structure or microservices. These patterns split your application into smaller, impartial pieces. Every module or provider can scale By itself with out impacting The full procedure.
Also, consider your database from working day a person. Will it require to deal with one million customers or merely 100? Pick the right sort—relational or NoSQL—based upon how your details will grow. Program for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential level is in order to avoid hardcoding assumptions. Don’t produce code that only is effective less than current disorders. Think about what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the databases slow down?
Use design and style designs that help scaling, like message queues or occasion-driven systems. These help your application cope with additional requests devoid of finding overloaded.
If you Create with scalability in mind, you're not just preparing for fulfillment—you happen to be minimizing potential head aches. A well-prepared process is simpler to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the proper Databases
Picking out the proper database is often a crucial A part of building scalable purposes. Not all databases are created the identical, and utilizing the Erroneous one can gradual you down or maybe result in failures as your application grows.
Start out by comprehension your information. Can it be very structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are definitely robust with interactions, transactions, and consistency. In addition they assist scaling techniques like examine replicas, indexing, and partitioning to handle additional site visitors and details.
When your data is much more adaptable—like user exercise logs, item catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured information and might scale horizontally extra very easily.
Also, take into consideration your study and publish styles. Have you been executing lots of reads with less writes? Use caching and browse replicas. Are you handling a weighty generate load? Consider databases that could tackle high compose throughput, or maybe party-dependent details storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to Imagine ahead. You may not want Innovative scaling capabilities now, but deciding on a databases that supports them means you won’t require to switch later on.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your facts dependant upon your entry designs. And generally observe databases general performance when you mature.
To put it briefly, the right databases depends on your application’s composition, velocity desires, And just how you be expecting it to improve. Acquire time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each little hold off provides up. Badly composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s essential to Create productive logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away everything needless. Don’t choose the most sophisticated Answer if a straightforward one particular functions. Keep the features brief, concentrated, and simple to check. Use profiling instruments to locate bottlenecks—sites the place your code will take much too prolonged to run or works by using a lot of memory.
Future, have a look at your database queries. These generally slow points down greater than the code alone. Make certain Each individual query only asks for the info you actually need to have. Steer clear of Pick out *, which fetches every thing, and as a substitute choose precise fields. Use indexes to speed up lookups. And stay away from accomplishing too many joins, In particular throughout huge tables.
For those who discover the exact same data currently being asked for again and again, use caching. Keep the outcome quickly utilizing instruments like Redis or Memcached and that means you don’t really have to repeat costly operations.
Also, batch your database functions after you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job good with 100 information may possibly crash if they have to take care of one million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when necessary. These methods enable your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage additional people plus more targeted visitors. If everything goes as a result of one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these equipment support keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across several servers. Rather than 1 server doing many of the do the job, the load balancer routes people to diverse servers depending on availability. This implies no one server receives overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it could be reused rapidly. When users ask for the identical information yet again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for quickly obtain.
two. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching cuts down database load, enhances speed, and can make your application more productive.
Use caching for things which don’t modify normally. And often be certain your cache is up to date when facts does change.
In a nutshell, load balancing and caching are simple but effective applications. With each other, they help your application handle a lot more people, stay quickly, and Get well from problems. If you plan to increase, you would like each.
Use Cloud and Container Instruments
To make scalable applications, you will need instruments that permit your application develop very easily. That’s wherever cloud platforms and containers are available. They offer you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess potential ability. When targeted traffic boosts, you could increase extra resources with just a few clicks or automatically using auto-scaling. When traffic more info drops, you can scale down to save money.
These platforms also offer products and services like managed databases, storage, load balancing, and stability applications. You may center on making your application as opposed to controlling infrastructure.
Containers are An additional important tool. A container offers your application and every little thing it must run—code, libraries, configurations—into one particular unit. This makes it quick to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your application works by using a number of containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it mechanically.
Containers also allow it to be straightforward to individual parts of your application into providers. You can update or scale sections independently, which can be perfect for functionality and reliability.
Briefly, utilizing cloud and container instruments implies you could scale quickly, deploy easily, and Recuperate immediately when troubles happen. If you need your application to expand without the need of limitations, start out utilizing these equipment early. They help you save time, minimize possibility, and assist you to keep centered on developing, not repairing.
Observe Every thing
In case you don’t observe your application, you gained’t know when items go Erroneous. Checking assists the thing is how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a crucial Section of setting up scalable systems.
Commence by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and solutions are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you obtain and visualize this data.
Don’t just keep track of your servers—check your app also. Control just how long it will require for people to load internet pages, how frequently glitches transpire, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a provider goes down, you must get notified right away. This aids you repair problems fast, normally right before people even observe.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it will cause actual harm.
As your application grows, targeted traffic and information maximize. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the proper applications in position, you stay on top of things.
In brief, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehending your process and ensuring it really works nicely, even stressed.
Last Feelings
Scalability isn’t just for massive firms. Even small applications need a powerful Basis. By creating thoroughly, optimizing wisely, and utilizing the right equipment, you could Construct applications that develop efficiently without breaking under pressure. Start out small, Consider significant, and Develop clever.